Week 1 – https://www.ntu.edu.sg/home/ehchua/programming/java/J3f_OOPExercises.html
Week 2: Builder pattern
https://www.vogella.com/tutorials/DesignPatternBuilder/article.html
https://www.geeksforgeeks.org/builder-pattern-in-java/
https://medium.com/@ajinkyabadve/builder-design-patterns-in-java-1ffb12648850
https://dzone.com/articles/design-patterns-builder
Task: create your own two project examples using this pattern (they don’t have to be large) .
Week 3: StringBuilder as example of Builder https://www.geeksforgeeks.org/string-vs-stringbuilder-vs-stringbuffer-in-java/
Task: create a project showing differences between StringBuilder and string.
Week 4: Prototype Pattern/Deep Copy
https://www.baeldung.com/java-pattern-prototype
https://refactoring.guru/design-patterns/prototype/java/example
Task1: Make class form UML Diagram and implement for it Prototype Pattern.
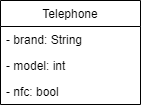
Task2: Implement DeepCopy for School class.
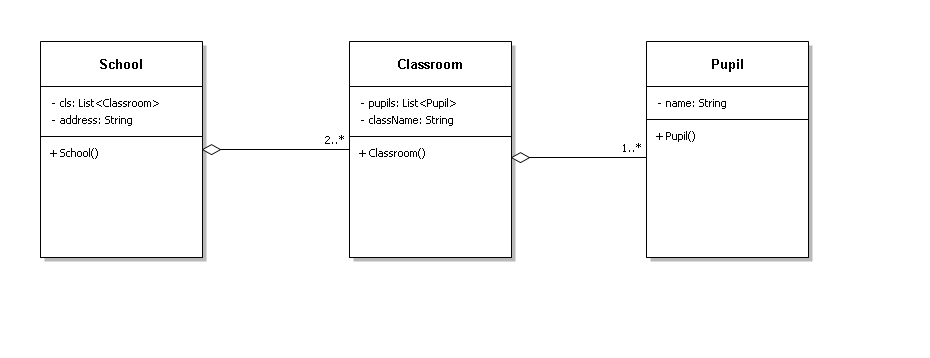
Week 5: Singleton Pattern
https://www.geeksforgeeks.org/singleton-class-java/
https://www.journaldev.com/1377/java-singleton-design-pattern-best-practices-examples
https://www.baeldung.com/java-singleton
Taks1: Implement the singleton pattern for the following class:
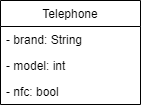
Task2: Modify the singleton pattern for any class to allow up to two instances.
Week 6: Abstract Factory, Factory Method
https://www.baeldung.com/java-abstract-factory-pattern
https://refactoring.guru/design-patterns/abstract-factory/java/example
https://www.tutorialspoint.com/design_pattern/factory_pattern.htm
https://www.javatpoint.com/factory-method-design-pattern
Task1: create two projects presenting both factories. The product is to be Pizza, and the parts – pizza ingredients.
Week 7: Adapter
https://www.geeksforgeeks.org/adapter-pattern/
https://www.tutorialspoint.com/design_pattern/adapter_pattern.htm
Task: implement Adapter Pattern for the following class:
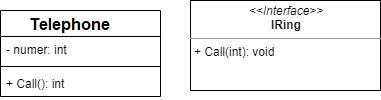
Week 8: Decorator& Facade Pattern
Decorator:
https://www.tutorialspoint.com/design_pattern/decorator_pattern.htm
https://refactoring.guru/design-patterns/decorator/java/example
Task: Using the Decorator pattern, create a project that simulates the operation of the bank.
Facade Pattern:
https://www.tutorialspoint.com/design_pattern/facade_pattern.htm
https://refactoring.guru/design-patterns/facade/java/example
Task: Using the Facade pattern, create a project that simulates the operation of the bank.
Week 9: Composite & Bridge Pattern
Composite:
https://www.baeldung.com/java-composite-pattern
https://www.geeksforgeeks.org/composite-design-pattern/
Bridge Pattern:
https://refactoring.guru/design-patterns/bridge/java/example
https://dzone.com/articles/bridge-design-pattern-in-java
Task: Prepare two projects illustrating both design patterns.
Week 10: Proxy & Flyweight Pattern
Flyweight
https://www.tutorialspoint.com/design_pattern/flyweight_pattern.htm
https://refactoring.guru/design-patterns/flyweight/java/example
Proxy
https://www.baeldung.com/java-proxy-pattern
https://www.baeldung.com/java-proxy-pattern
Task: for the class Telephone prepare two projects illustrating both design patterns.
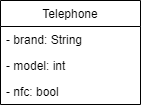